ImqCICSBridgeHeader C++ class
This class encapsulates specific features of the MQCIH data structure.
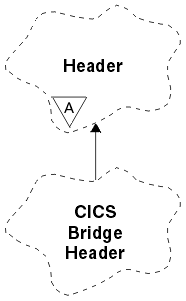
Objects of this class are used by applications that send messages to the CICS bridge through IBM MQ for z/OS .
- Object attributes
- Constructors
- Overloaded ImqItem methods
- Object methods (public)
- Object data (protected)
- Reason codes
- Return codes
Object attributes
- ADS descriptor
- Send/receive ADS descriptor. This is set using MQCADSD_NONE. The initial value is MQCADSD_NONE. The following additional values are possible:
- MQCADSD_NONE
- MQCADSD_SEND
- MQCADSD_RECV
- MQCADSD_MSGFORMAT
- attention identifier
- AID key. The field must be of length MQ_ATTENTION_ID_LENGTH.
- authenticator
- RACF password or passticket. The initial value contains blanks, of length MQ_AUTHENTICATOR_LENGTH.
- bridge abend code
- Bridge abend code, of length MQ_ABEND_CODE_LENGTH. The initial value is four blank characters. The value returned in this field is dependent on the return code. See Table 1 for more details.
- bridge cancel code
- Bridge abend transaction code. The field is reserved, must contain blanks, and be of length MQ_CANCEL_CODE_LENGTH.
- bridge completion code
- Completion code, which can contain either the IBM MQ completion code or the CICS EIBRESP value. The field has the initial value of MQCC_OK. The value returned in this field is dependent on the return code. See Table 1 for more details.
- bridge error offset
- Bridge error offset. The initial value is zero. This attribute is read-only.
- bridge reason code
- Reason code. This field can contain either the IBM MQ reason or the CICS EIBRESP2 value. The field has the initial value of MQRC_NONE. The value returned in this field is dependent on the return code. See Table 1 for more details.
- bridge return code
- Return code from the CICS bridge. The initial value is MQCRC_OK.
- conversational task
- Whether the task can be conversational. The initial value is MQCCT_NO. The following additional values are possible:
- MQCCT_YES
- MQCCT_NO
- cursor position
- Cursor position. The initial value is zero.
- facility keep time
- CICS bridge facility release time.
- facility like
- Terminal emulated attribute. The field must be of length MQ_FACILITY_LIKE_LENGTH.
- facility token
- BVT token value. The field must be of length MQ_FACILITY_LENGTH. The initial value is MQCFAC_NONE.
- function
- Function, which can contain either the IBM MQ call name or the CICS EIBFN function. The field has the initial value of MQCFUNC_NONE, with length MQ_FUNCTION_LENGTH. The value returned in this field is dependent on the return code. See Table 1 for more details.
The following additional values are possible when function contains an IBM MQ call name:
- MQCFUNC_MQCONN
- MQCFUNC_MQGET
- MQCFUNC_MQINQ
- MQCFUNC_NONE
- MQCFUNC_MQOPEN
- MQCFUNC_PUT
- MQCFUNC_MQPUT1
- get wait interval
- Wait interval for an MQGET call issued by the CICS bridge task. The initial value is MQCGWI_DEFAULT. The field applies only when uow control has the value MQCUOWC_FIRST. The following additional values are possible:
- MQCGWI_DEFAULT
- MQWI_UNLIMITED
- link type
- Link type. The initial value is MQCLT_PROGRAM. The following additional values are possible:
- MQCLT_PROGRAM
- MQCLT_TRANSACTION
- next transaction identifier
- ID of the next transaction to attach. The field must be of length MQ_TRANSACTION_ID_LENGTH.
- output data length
- COMMAREA data length. The initial value is MQCODL_AS_INPUT.
- reply-to format
- Format name of the reply message. The initial value is MQFMT_NONE with length MQ_FORMAT_LENGTH.
- start code
- Transaction start code. The field must be of length MQ_START_CODE_LENGTH. The initial value is MQCSC_NONE. The following additional values are possible:
- MQCSC_START
- MQCSC_STARTDATA
- MQCSC_TERMINPUT
- MQCSC_NONE
- task end status
- Task end status. The initial value is MQCTES_NOSYNC. The following additional values are possible:
- MQCTES_COMMIT
- MQCTES_BACKOUT
- MQCTES_ENDTASK
- MQCTES_NOSYNC
- transaction identifier
- ID of the transaction to attach. The initial value must contain blanks, and must be of length MQ_TRANSACTION_ID_LENGTH. The field applies only when uow control has the value MQCUOWC_FIRST or MQCUOWC_ONLY.
- UOW control
- UOW control. The initial value is MQCUOWC_ONLY. The following additional values are possible:
- MQCUOWC_FIRST
- MQCUOWC_MIDDLE
- MQCUOWC_LAST
- MQCUOWC_ONLY
- MQCUOWC_COMMIT
- MQCUOWC_BACKOUT
- MQCUOWC_CONTINUE
- version
- The MQCIH version number. The initial value is MQCIH_VERSION_2. The only other supported value is MQCIH_VERSION_1.
Constructors
- ImqCICSBridgeHeader( );
- The default constructor.
- ImqCICSBridgeHeader( const ImqCICSBridgeHeader & header );
- The copy constructor.
Overloaded ImqItem methods
- virtual ImqBoolean copyOut( ImqMessage & msg );
- Inserts an MQCIH data structure into the message buffer at the beginning, moving existing message data further along, and sets the message format to MQFMT_CICS.
See the parent class method description for more details.
- virtual ImqBoolean pasteIn( ImqMessage & msg );
- Reads an MQCIH data structure from the message buffer. To be successful, the encoding of the msg object must be MQENC_NATIVE. Retrieve messages with MQGMO_CONVERT to MQENC_NATIVE. To be successful, the ImqMessage format must be MQFMT_CICS.
See the parent class method description for more details.
Object methods (public)
- void operator = ( const ImqCICSBridgeHeader & header );
- Copies instance data from the header, replacing the existing instance data.
- MQLONG ADSDescriptor( ) const;
- Returns a copy of the ADS descriptor.
- void setADSDescriptor( const MQLONG descriptor = MQCADSD_NONE );
- Sets the ADS descriptor.
- ImqString attentionIdentifier( ) const;
- Returns a copy of the attention identifier, padded with trailing blanks to length MQ_ATTENTION_ID_LENGTH.
- void setAttentionIdentifier( const char * data = 0 );
- Sets the attention identifier, padded with trailing blanks to length MQ_ATTENTION_ID_LENGTH. If no data is supplied, resets attention identifier to the initial value.
- ImqString authenticator( ) const;
- Returns a copy of the authenticator, padded with trailing blanks to length MQ_AUTHENTICATOR_LENGTH.
- void setAuthenticator( const char * data = 0 );
- Sets the authenticator, padded with trailing blanks to length MQ_AUTHENTICATOR_LENGTH. If no data is supplied, resets authenticator to the initial value.
- ImqString bridgeAbendCode( ) const;
- Returns a copy of the bridge abend code, padded with trailing blanks to length MQ_ABEND_CODE_LENGTH.
- ImqString bridgeCancelCode( ) const;
- Returns a copy of the bridge cancel code, padded with trailing blanks to length MQ_CANCEL_CODE_LENGTH.
- void setBridgeCancelCode( const char * data = 0 );
- Sets the bridge cancel code, padded with trailing blanks to length MQ_CANCEL_CODE_LENGTH. If no data is supplied, resets the bridge cancel code to the initial value.
- MQLONG bridgeCompletionCode( ) const;
- Returns a copy of the bridge completion code.
- MQLONG bridgeErrorOffset( ) const ;
- Returns a copy of the bridge error offset.
- MQLONG bridgeReasonCode( ) const;
- Returns a copy of the bridge reason code.
- MQLONG bridgeReturnCode( ) const;
- Returns the bridge return code.
- MQLONG conversationalTask( ) const;
- Returns a copy of the conversational task.
- void setConversationalTask( const MQLONG task = MQCCT_NO );
- Sets the conversational task.
- MQLONG cursorPosition( ) const ;
- Returns a copy of the cursor position.
- void setCursorPosition( const MQLONG position = 0 );
- Sets the cursor position.
- MQLONG facilityKeepTime( ) const;
- Returns a copy of the facility keep time.
- void setFacilityKeepTime( const MQLONG time = 0 );
- Sets the facility keep time.
- ImqString facilityLike( ) const;
- Returns a copy of the facility like, padded with trailing blanks to length MQ_FACILITY_LIKE_LENGTH.
- void setFacilityLike( const char * name = 0 );
- Sets the facility like, padded with trailing blanks to length MQ_FACILITY_LIKE_LENGTH. If no name is supplied, resets facility like the initial value.
- ImqBinary facilityToken( ) const;
- Returns a copy of the facility token.
- ImqBoolean setFacilityToken( const ImqBinary & token );
- Sets the facility token. The data length of token must be either zero or MQ_FACILITY_LENGTH. It returns TRUE if successful.
- void setFacilityToken( const MQBYTE8 token = 0);
- Sets the facility token. token can be zero, which is the same as specifying MQCFAC_NONE. If token is nonzero it must address MQ_FACILITY_LENGTH bytes of binary data. When using predefined values such as MQCFAC_NONE, you might need to make a cast to ensure a signature match. For example, (MQBYTE *)MQCFAC_NONE.
- ImqString function( ) const;
- Returns a copy of the function, padded with trailing blanks to length MQ_FUNCTION_LENGTH.
- MQLONG getWaitInterval( ) const;
- Returns a copy of the get wait interval.
- void setGetWaitInterval( const MQLONG interval = MQCGWI_DEFA
- Sets the get wait interval.
- MQLONG linkType( ) const;
- Returns a copy of the link type.
- void setLinkType( const MQLONG type = MQCLT_PROGRAM );
- Sets the link type.
- ImqString nextTransactionIdentifier( ) const ;
- Returns a copy of the next transaction identifier data, padded with trailing blanks to length MQ_TRANSACTION_ID_LENGTH.
- MQLONG outputDataLength( ) const;
- Returns a copy of the output data length.
- void setOutputDataLength( const MQLONG length = MQCODL_AS_INPUT );
- Sets the output data length.
- ImqString replyToFormat( ) const;
- Returns a copy of the reply-to format name, padded with trailing blanks to length MQ_FORMAT_LENGTH.
- void setReplyToFormat( const char * name = 0 );
- Sets the reply-to format, padded with trailing blanks to length MQ_FORMAT_LENGTH. If no name is supplied, resets reply-to format to the initial value.
- ImqString startCode( ) const;
- Returns a copy of the start code, padded with trailing blanks to length MQ_START_CODE_LENGTH.
- void setStartCode( const char * data = 0 );
- Sets the start code data, padded with trailing blanks to length MQ_START_CODE_LENGTH. If no data is supplied, resets start code to the initial value.
- MQLONG taskEndStatus( ) const;
- Returns a copy of the task end status.
- ImqString transactionIdentifier( ) const;
- Returns a copy of the transaction identifier data, padded with trailing blanks to the length MQ_TRANSACTION_ID_LENGTH.
- void setTransactionIdentifier( const char * data = 0 );
- Sets the transaction identifier, padded with trailing blanks to length MQ_TRANSACTION_ID_LENGTH. If no data is supplied, resets transaction identifier to the initial value.
- MQLONG UOWControl( ) const;
- Returns a copy of the UOW control.
- void setUOWControl( const MQLONG control = MQCUOWC_ONLY );
- Sets the UOW control.
- MQLONG version( ) const;
- Returns the version number.
- ImqBoolean setVersion( const MQLONG version = MQCIH_VERSION_2 );
- Sets the version number. It returns TRUE if successful.
Object data (protected)
- MQLONG olVersion
- The maximum MQCIH version number that can be accommodated in the storage allocated for opcih.
- PMQCIH opcih
- The address of an MQCIH data structure. The amount of storage allocated is indicated by olVersion.
Reason codes
- MQRC_BINARY_DATA_LENGTH_ERROR
- MQRC_WRONG_VERSION
Return codes
Return Code | Function | CompCode | Reason | Abend Code |
---|---|---|---|---|
MQCRC_OK | ||||
MQCRC_BRIDGE_ERROR | MQFB_CICS | |||
MQCRC_MQ_API_ERROR | IBM MQ call name | IBM MQ CompCode | IBM MQ Reason | |
MQCRC_BRIDGE_TIMEOUT | IBM MQ call name | IBM MQ CompCode | IBM MQ Reason | |
MQCRC_CICS_EXEC_ERROR | CICS EIBFN | CICS EIBRESP | CICS EIBRESP2 | |
MQCRC_SECURITY_ERROR | CICS EIBFN | CICS EIBRESP | CICS EIBRESP2 | |
MQCRC_PROGRAM_NOT_AVAILABLE | CICS EIBFN | CICS EIBRESP | CICS EIBRESP2 | |
MQCRC_TRANSID_NOT_AVAILABLE | CICS EIBFN | CICS EIBRESP | CICS EIBRESP2 | |
MQCRC_BRIDGE_ABEND | CICS ABCODE | |||
MQCRC_APPLICATION_ABEND | CICS ABCODE |